React Interview Q&A
TOP 10 Interview Question in React
Table of Contents
Q1 : What is React? What is the Role of React in Software Development ?
Answer: 3 points need to remember about React :
- React is open source Javascript Libraby.
- React is used for user interfaces.
- React simplifies the creation of SPA (Single Page Application) by using Reusable Component.
Q2 : What are the Key Features of React ?
Answer: There are 7 Key Features of React.
- Virtual DOM
- Component based architecture
- Reusability & Composition
- JSX(Java Script & XML)
- Declarative Syntax
- Community & Ecosystem
- React Hook
Q3 : What is Virtual DOM? Difference between DOM and Virtual DOM?
Answer : First we discuss about real DOM. whenever the user will do any changes in application basically we are updating the real DOM right, now what is Virtual DOM and why do we need it?
First of all DOM is a general and real concept in all the browsers. Without DOM our application can’t interact or handle JavaScript but Virtual DOM is specific for react only and it is not mandatory. It is developed by Facebook for improve the speed of the application.
Basically there is a performance problem in Real DOM. The problem is even if a user makes very small change in web application in the browser even then the whole layout will render in the DOM. For example, If a user change only one element in title of html even then browser will re-render or refresh whole layout of the entire page. It will very time consuming, that time consumption will increase the loaded time of the page and that will increase the speed and performance problem. To solve the problem we use Virtual DOM.
Learn React In 2024 – A Step-By-Step Guide
In React application, React user open react based website in there browser then in the background React library will make and exact copy of the DOM and show that copy to the front of user. So this exact copy of DOM is Virtual DOM. When the user make some changes to the elements in html it looks like user is interacting with real DOM but actually the user make changes in Virtual DOM.
The specialty of Virtual DOM is if the user makes some changes any element in HTML now Virtual DOM not render whole virtual DOM for small changes. Only the small specific part of the virtual DOM will be updated and In background React algorithm of React Library will keep comparing the changes between Virtual DOM and Real DOM and whatever change has made by user in Virtual DOM only those changes will be updated in Real DOM.
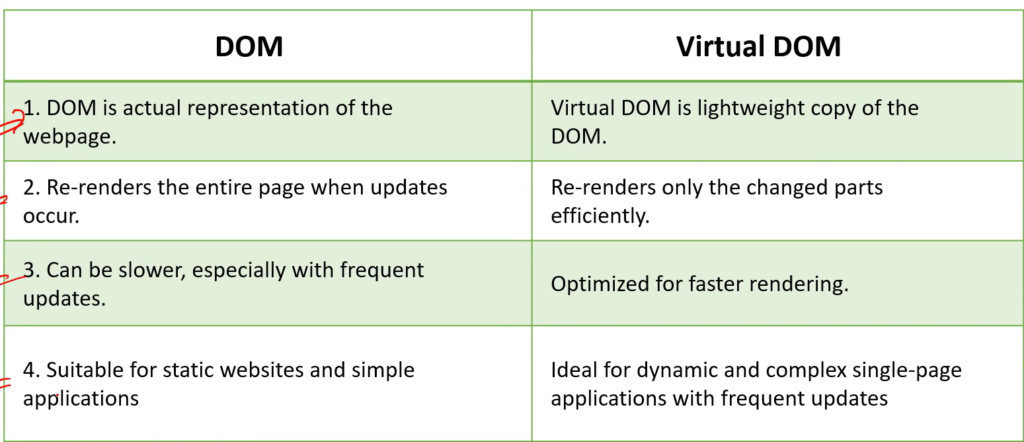
Q4 : What are React Components? What are main elements of it?
Answer : In React, a component is a reusable building block for creating user interfaces. It can be either functional or class-based.
Main element of React :
- Import
- Export
Q5 : What are the 5 advantage of React ?
Answer: Advantage of React :
- Simple to build Single Page Application by using Component.
- React is cross platform and open source (Free to Use).
- React is lightweight and very fast(Virtual DOM).
- Large community and ecosystem.
- Testing is easy.
Q6. What is the role of JSX in React?
Answer : JSX stands for JavaScript XML. The key point of JSX are :
- JSX is used by React to write HTML-like code within JavaScript.
- JSX makes easier to write and add HTML in React.
- JSX uses camelcase notification for naming HTML attribute. For example, username in HTML is used as userName in JSX.
- To insert a large number of HTML we have to write it in parenthesis i.e, ().
Q7. What is SPA(Single Page Application)?
Answer : A single Page Application(SPA) is a web application that is designed to be displayed as a single, static page. As the user clicks link and interact with the page of the website, subsequent content is loaded dynamically. The result is more fluid and faster without any page refresh.
Q8. What difference between Declarative and Imperative Syntax?
Answer :
Imperative
- Procedural Programming Paradigm
- Object Oriented Programming
- Parallel Processing Approach
- The User is allowed to make decisions and commands to the compiler.
- Variable can be mutable
- It provides step-by-step DOM mutations until we reach desired UI.
Imperative syntax(non-React) using JavaScript
function App(){ const element = document.createElement(“h1”); element.textContent = “Hello World”; document.body.appendChild(element); }
Declarative
- Login Programming Paradigm
- Functional Programming
- Database Processing Approach
- A compiler is allowed to make decisions.
- Variable are typically immutable.
- We doesn’t provide step-by-step instructions to reach the desired UI. Just tell React what to render in component’s logic, and React will figure out how best to display it to the user.
Declarative syntax using JSX
function App(){ return <h1> Hello World </h1>; }
Q9. How to pass data from Parent Component to Child Component in React?
Answer : In React, “props” are a mechanism for passing data from parent component to child component. Props are immutable means if the data are set by the parent component, they can not be changed by child component. The child component can only use it but should not modify.
// Parent component
function ParentComponent() {
const message = "Welcome from parent!";
return <ChildComponent greeting={message} />;
}
// Child component
function ChildComponent(props) {
return <p>{props.greeting}</p>;
}
Q10. What are React Hooks? Why Hooks are introduced in React?
Answer : Before React version 16.8, functional component can’t handle state, logic and lots of other React features and we only used them for rendering very simple components to the UI. To resolve this problem React Hooks are introduced.
Each React Hooks name is prefixed with the word “use”. For example useState and useEffect.
Related Topics